Programming
- lukepat74
- 1 maj 2014
- 8 minut(y) czytania

Up till now, it's been an extreme challenge that could be mastered by specialists only. Today, we're gonna encourage You that it's not !
A proposed language perfect for beginners:
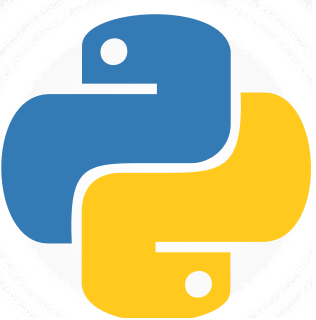
Python - a perfect language for beginners, easy to learn. It comes with libraries - additions to the language that contain various additional commands. You gain access to them in Your program writing :
from <name> import *
and even create your own ones!
example code. It's a guessing game:
from random import *
b = randint(0,10)
a = int(input("What number do You guess?(0 - 10)") )
if a > b:
print("too great")
if a < b:
print("too small!")
if a == b:
print("yes!")
while a != b:
a = int(input("What number do You guess?(0 - 10)"))
if a > b:
print("too great")
if a < b:
print("too small!")
print("Guessed!!!")
Result:
First, the console asks You what number to choose.
If it's correct, then writes "Yes! Guessed!", but if not, tells You whether the guessed number is too big or too small untill the guess is correct.
Excmaimation:
print(<text>) dispays a chosen text in the console.
randint(<from>, <up to>) - a command from the random library that picks a random number of a value from the value of the first parameter, up to the value of the second one.
while <condition>:
<all the code for it to be executed has to be indented>
Repeats all the code in it until a contition is true.
if <condition>:
<all the code for it to be executed has to be indented>
Executes all the code in it if a contition is true.
a = input(<what to write>) writes the value of the parameter in the console and lets the user write something, but as there's int(input()), the thing You input can only be a number, because "int()" comes from "integer" which is a number. The value of what You've written is "hidden" in the variable "a". Other charakters are "str()" for "string" which is all charakters, but always comes with quotes "<string>". The other one is float(). It's just a float number.
Necessary!
What is a parameter?
You know the command "print". In fact, it is a built-in function.
Creating a function is basically creating Your command.
The function print has one parameter: what to display.
You enter its value between the brackets.
Most functions have got many parameters, so You divide them using commas.
In conclusion, a parameter is a built-in, only for a certain fuction variable. Its value is not entered in the function. You, when You launch a function (e.g writing: print("amazing! ") launches the command(built-in fuction print)) have to set the parameter's value. In the given example, the parameter's value is "amazing!".
More advanced stuff:
lists.
A list is a group of variables or values. It can containg words(strings), values(integers) and variables.
example:
b = 5
a = ["pineapple",100,b]
This list contains a string "pineapple", an integer and a variable.
You can display what's inside them.
print(a[0:])
This displays all the content of the list a from its
first item (index: 0) till its end.
print(a)
Writing
print(a[0])
would display the first item of a. The same You can do with variables.
a = "flamingo"
print(a[1])
Result:
'l'
print(a[:3])
Result:
'fla'
List functions. If You want to add an item to the last position of the list we use append(<what to add>).
However, You can only use this and all the rest commands shown here if You create the list writing (unless it has got some items inside in the definition):
<list name> = list()
<list name>.append()
To add an item to a list at a specified position, we use insert. It's the same as append, but it has the second parameter: where to add an item.
Example:
a = ["pizza","flower","techno-logical"]
a.insert(2,"another")
print(a)
Other similar things:
A tuple is a list where You cannot change its conetnt's value. For exaple, You can't write such a programme:
a = ("a","b")
a = ("b","a")
You define a tuple with its name and the content is in the round brackets.
A dictionary.(Check on an under-development site: More programming).
More from Python and other languages is on an under-development site: More programming).
Like it? There is plenty of interactive tutorials, videos or books that can develop Your interest or satisfy curiosity! If You don't understand, these are especially for You!
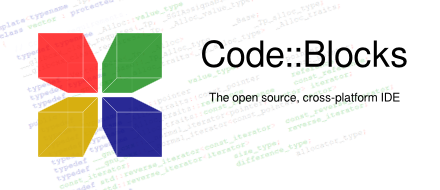
Another programming language is C++
It is faster than Python, although it requires a lot more work to create a programme. C++ is more dedicated for those, who already know the basics of programming, rather than for true beginnners.
It is best to programme on a coding platform called "Code Blocks" (in my opinion). Below is a simple code that adds two numbers that the user writes in:
#include <iostream>
using namespace std;
int main()
{
int a = 0;
int b = 0;
cin >> a;
cout << " + ";
cin >> b;
cout << " = " << a+b << endl;
return 0;
}
So, let's talk about the code.
The "#include <iostream>" imports a library called "input-output stream", which allows the user and computer to write and allows the computer and player read it.
The "int main() {}" is the main function, where the code goes (with some exceptions).
The "int <name_of_variable> = <value>" allows to create a whole number and name it.
The "cin >> <the_variable>" allows the user to write a number, which the variable will take as a value.
The "cout << <what_to_write>" allows the computer to write something. If the <what_to_write> is in the "", It writes exacly what given. You can also write the name of a variable there, and the computer will write the value of the variable.
NOTE: you have to write a ";" sign after each line (except defining libraries).
Now, let's make the same thing happen as the first programme in the Python section.
code 2:
using namespace std;
int main() {
int input = 0; int ctr = 1; srand(time(NULL)); int a = rand()%10+1;
cout << "guess the number I am thinking about (1-10)" << endl; while(input!=a) { cin >> input;
if(input==a) {
cout << " You guessed it! it's: " << a << "." << " It took you " << ctr << " tries!" << endl;
break; }
if(input<a) { cout << "Greater!" << endl; ctr++; }
if(input>a) { cout << "Smaller!" << endl; ctr++; }
} }
The "#include <random>" imports a library that enables a pseudo-random choice of a number.
The "srand(time(NULL))" makes the random number algorithm to be based on time.
The "rand()%<highest_number>+<lowest_number>" allows you to generate a rendom number.
The "while(<condition>) {<what_to_do}" is a loop that does the instructions below while the condition is true.
The "break" command stops the loop it is in.
The "<variable1>==<variable2>" is checking an equality, while the "<variable>=<value>" is changing the value to and the "<variable1>!=<variable2>" means not equal.
The "<variable>++" adds one to the valuable. Another form of it is "<variable>+=<value>",which increases the variable by the value.
Before we jump to the next project, let's learn what are functions.
They have the following layout:
<type><name>(<arguments>)
{}. They can only exist outside of the main function. They can have all the types that are used for variables, along with a void type, which does not return anything. Inside the brackets, you put all the arguments. To call the function, inside the main function, you write
<function_name>
(<argument_values>)
Inside the function's {}, you have to "tell" the function what to do with the values that will later be given in the main.
Below is a programme using vectors and the bubble sorting algorythm. But firstly, let's go over what are vectors and the bubble sorting algorythm.
Vectors show what direction and how far an object travels in the following way: [x_value;y_value;z_value], but can also have thousands of elements or be used as arrays.
Bubble sorting is an algorythm of sorting numbers. It takes a number and compares it with the next one. If the next number is smaller, the numbers are swapped. If not, they are left as they are and the next number is compared in the same way and so on. Below is a video of how it works:
(It's only worth watching until 1:48, don't watch it further)
So, let's go to the code:
using namespace std;
void bubble(vector <int> v) { }
for(int i = 0;i<v.size();i++) { cout << setw(4) << v.at(i) << " "; }
cout << endl << setfill('-') << setw(50) << endl; cout << setfill(' ') << endl;
for(int n = 0;n<v.size();n++) { for(int i = n%2;i<v.size()-1-(n%2);i++) { if(v.at(i)>v.at(i+1)) { swap(v.at(i),v.at(i+1)); }
}
}
for(int i = 0;i<v.size();i++) { cout << setw(4) << v.at(i) << " "; }
}
int main() {
srand(time(NULL)); vector <int> v1;
int N = 0;
cin >> N;
cout << endl;
for(int i = 0;i<=N;i++)
{
v1.push_back(rand()%N);
}
bubble(v2);
return 0; }
The <vector> library allows vectors to be used, along with the commands: at(), swap(), push_back(), size().
The <iomanip> library allows the programmer to manipulate with the layout of the text written in the console.
The for(<type_of_variable><name>=<value>;<argument>;<changes_to_variable>){} is a loop that seems to be tricky, but is very useful. let's look at an example from the code:
for(int i = 0;i<=N;i++)
{
v1.push_back(rand()%N);
}
As you can see, in the brackets, you have to declare a value.Then, give an argument. while it is true, the loop will repeat its code inside the {}.In the end, write what will happen to the value each time the loop repeats itself.
The <vector_name>.push_back (<element_value>) creates another element to the vector, setting it at the value given.
The<vector_name>.at(<element>) is generally used to doing something to one element of a vector.
The<vector_name>.size is the number of elements the vector has.
The <swap>(<element1>,<element2>) swaps two elements in a vector, and the at command has to be used in the place of element1 and element2.
The setw(<number>) makes all the written numbers have a <number> amount of spaces. If the spaces are not filled, they remain on the front. For example:
Without setw(3):
96 97 98 99 100 101 102 103
104 105 106 107 108 108 110
With setw(3):
96 97 98 99 100 101 102 103
104 105 106 106 107 108 109 110
Java - The future.

Although it's a more difficult than C and all its strains (C#, C++), You can do much more with it - mobile games for Android, iOS or Microsoft phone, but also e.x for all versions of xBox and PS.
It's sometimes even faster than C. That's because Java uses a compiler as well, but the main reason is that Java is multithreaded.
It means that Java programmes are divided into classes - small sub-programmes - saying easier. They are all separatelly compiled. It means that Your programme or game can do multiply things at a time which makes the it even faster too. However, we don't recommend Java for beginners.
Example programme:
import java.util.Random; public class silly_sentences { public static String[] nouns = {"bicycle","Patrick", "Luke","Matt","plane", "train","bed","car","room","chair","nothing","void","destruction","Nick","Nina","pizza","shoe","shop"}; public static String[] verbs = {"eats a
","drinks a", "touches a","burns a","kicks a", "melts a","anihilates","hated","loves","enters a dimension of","dances","explodes","makes fun of a","nibbles","bites","ripps","kills","puts on a"}; public static void choice(String[] table) { int len = table.length; Random index = new Random(); System.out.print(table[index.nextInt(len)]+" "); } public static void main(String[] args) { choice(nouns); choice(verbs); choice(nouns); } } Explaination: public static void <name>(<optional parameter/s>)
{
enter some code
}
It is a function. A function is basically a command created by the coder.
Everything it does is situated between the two {} brackets.
Whereas
public static void main(String[] args)
{
}
is a constructor - a function that is necessary for the program to run. All the startup code place in there.
<optional parameter/s> Parameters are variables that you set when launching the function ( exFunction(<set parameters' value here>); ). They can be integers, strings, floats, etc. ,
Static - Functions can also be set like this : public void smth(){}. However, we say they're non-static then, because they don't have "static" after "public". Although, the difference between static functions and non-static ones is bigger and we shall only tell the basic one. It is that You can neither launch a static function inside a non-static one, nor You can launch a non-static function inside a static one. That's why we recommend You to use functions of only one type.
public String[] nouns = {}; Is an Array.It's just like a list in Python. However, in Java there are no tuples and dictionaries.
Comments