More Arduino
- lukepat74
- 21 lut 2017
- 2 minut(y) czytania
Last time, the Arduino program wasn't that great, as it used a built-in L.E.D diode on the Arduino board.
Let's do something more advanced!
OK. It's basically the same thing as the last time, but with a L.E.D diode on the breadboard.
How an Arduino-Breadboard circuit looks like:
For the electricity to go through, You will always have to plug GND(Arduino board) which is the '-' current to Your circuit.(except Your selected pin or pins). Except GND You also have 5v and 3.5v on the Arduino board. Those are '+'.
A photo to help You:
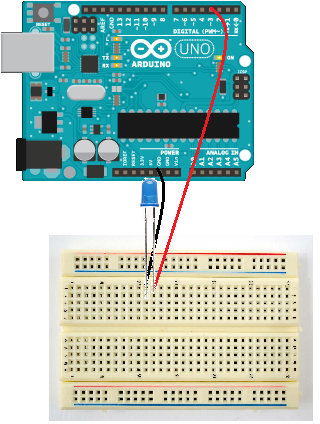
Here's the code. Look how similar it is to the first one!
void setup() { pinMode(3,OUTPUT); } void loop() { digitalWrite(3,HIGH); delay(1000); digitalWrite(3,LOW); delay(1000); }
Next one (something bigger!):
This circuit contains 3 L.E.D diodes that flash one after another.
Video:
Photo:
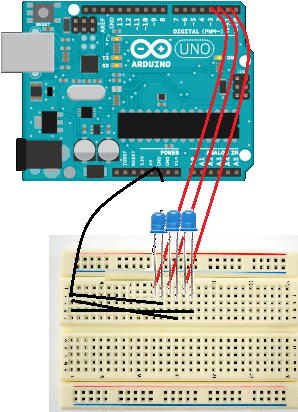
Code:
void setup() { for(int i=1;i<4;i++) { pinMode(i,OUTPUT); } } void loop() { for(int i=1;i<4;i++) { digitalWrite(i,HIGH); delay(500); digitalWrite(i,LOW); delay(500); } for(int i=4;i>1;i--) { digitalWrite(i,HIGH); delay(500); digitalWrite(i,LOW); delay(500); } }
What's new here?
For loops.
Now, You know one type of loop - whi le loop. It runs a code inside it until a condition is true. For loop repeats a code for a certain amount of time. First, You set a variable in the loop. Here,it's an integer "i". Then You set untilwhen the code inside the loop is repeated. The last step is setting the increasment and decreasment of the loop's variable. Logically, is You first set the loop's variable e.g. to 0 and the
condition < 10, in the third parameter the loop's variable will have to increase, not decrease.
Note that You can modify the loop's variable inside the loop or use it in all purposes, but not outside the loop.
That is why we've written pinMode(i,OUTPUT); inside a for loop. We didn't have to write pinMode(); three times. The number of the activated pin is just the value of the loop's variable. The same thing happens to digitalWrite();
Another one. It shows the use of digital pins with the analog property and analogWrite(); function.
What are functions and parameters? Visit the 'programming post'.
Comments